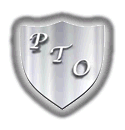
ProTech-Online.com
This is a Linux C++ code example of accessing the Zeus GPIO.
#include <iostream>
#include <sstream>
#include <string>
#define usleep(x) usleep((int)((x)
* 1000000))
using namespace std;
class vgpio {
public:
vgpio();
~vgpio();
string getgpio();
bool doorsensors();
bool tanksensor();
void popdoor();
};
vgpio::vgpio() {
system("i2c-regs 0x20 0x6
0xfe");
system("i2c-regs 0x20 0x2
0xff");
cout << "GPIO initialized, Value = " << getgpio() << endl;
}
vgpio::~vgpio()
{
}
string vgpio::getgpio(){
FILE
*file;
char str[1024];
string str_buffer;
if ( !(file = popen("i2c-regs 0x20
0x0","r")) ) {
// If file is NULL
perror("i2c-regs error");
exit(1);
}
while ( fgets( str, sizeof str, file)) {
str_buffer.append(str);
}
pclose(file);
return str_buffer;
}
bool vgpio::doorsensors(){
string result;
int x,y;
bool on;
result = getgpio();
result = result.substr(2,2);
istringstream conv(result);
conv >> hex >> x;
y = x |
15;
on = (y == 15) ? true : false;
return on;
}
bool vgpio::tanksensor(){
string result;
int x,y;
bool on;
result = getgpio();
result = result.substr(2,2);
istringstream conv(result);
conv >> hex >> x;
y = x
| 251;
on = (y == 251) ? true : false;
return on;
}
void vgpio::popdoor(){
system("i2c-regs 0x20 0x2
0xfe");
usleep(5);
system("i2c-regs 0x20 0x2
0xff");
}
Copyright © 2013 ProTechs-Online.com; All rights reserved.