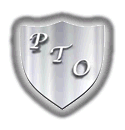
ProTech-Online.com
This is a Linux C++ code example of accessing the Viper GPIO.
#include <iostream>
#include <sstream>
#include <string>
#include <fcntl.h>
#define usleep(x) usleep((int)((x)
* 1000000))
using namespace std;
class vgpio {
public:
vgpio();
~vgpio();
string getgpio();
bool doorsensors(int);
bool doorsensor(int);
bool tanksensor(string);
void CardReaderOn();
void popdoor(string);
void popdoors();
};
vgpio::vgpio() {
system("pxaregs GPSR0_20 1");
system("pxaregs GPSR0_26 1");
cout << "GPIO initialized, Value = " << getgpio() << endl;
}
vgpio::~vgpio()
{
}
string vgpio::getgpio(){
FILE
*file;
char str[1024];
string str_buffer;
int position;
if ( !(file = popen("devmem2 0x14500000 b","r"))
) {
// If file is NULL
perror("devmem2 error");
exit(1);
}
while ( fgets( str, sizeof str, file)) {
str_buffer.append(str);
}
pclose(file);
position = str_buffer.rfind("0x");
str_buffer = str_buffer.substr(position, 4);
return str_buffer;
}
bool vgpio::doorsensors(int cages){
bool on;
int c1, c2;
int sensors_status;
string result;
result = getgpio();
result = result.substr(2,2);
istringstream conv(result);
conv >> hex >> sensors_status;
switch(cages){
case 1:
c1
= sensors_status & 48;
on = (c1 != 48) ? true : false;
break;
case 2:
c1 = sensors_status & 48;
c2
= sensors_status & 192;
on = (c1 != 48 && c2 != 192) ? true : false;
break;
default:
on = false;
break;
}
return on;
}
bool vgpio::tanksensor(string cage){
bool on;
int t;
int sensors_status;
string result;
result = getgpio();
result = result.substr(2,2);
istringstream conv(result);
conv >> hex >> sensors_status;
if (cage == "1"){
t = sensors_status & 16;
on = (t != 16) ? true : false;
}
if (cage == "2"){
t = sensors_status & 64;
on = (t != 64) ? true : false;
}
return on;
}
void vgpio::popdoor(string cage){
if (cage == "1"){
cout << "pop cage1" << endl;
system("pxaregs GPCR0_21 1");
usleep(5);
system("pxaregs GPSR0_21 1");
}
if (cage == "2"){
cout << "pop cage2" << endl;
system("pxaregs GPCR0_22 1");
usleep(5);
system("pxaregs GPSR0_22 1");
}
}
void vgpio::CardReaderOn(){
cout << "turning card reader on" << endl;
system("pxaregs GPCR0_26 1");
}
void vgpio::popdoors(){
cout << "pop all doors" << endl;
system("pxaregs GPCR0_21 1");
usleep(5);
system("pxaregs GPSR0_21 1");
usleep(5);
system("pxaregs GPCR0_22 1");
usleep(5);
system("pxaregs GPSR0_22 1");
}
bool vgpio::doorsensor(int cages){
bool on;
int c;
int sensors_status;
string result;
result = getgpio();
result = result.substr(2,2);
istringstream conv(result);
conv >> hex >> sensors_status;
switch(cages){
case 1:
c = sensors_status & 48;
on = (c != 48) ? true : false;
break;
case 2:
c = sensors_status & 192;
on = (c != 192) ? true : false;
break;
default:
on = false;
break;
}
return on;
}
Copyright © 2013 ProTechs-Online.com; All rights reserved.