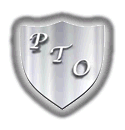
ProTech-Online.com
This is a Linux C++ code example of printing to a Hengstler C-56 Thermal Printer.
#include <termios.h>
#include <stdio.h>
#include <fcntl.h>
#include <sys/ioctl.h>
#include <ctime>
#include <string>
#define usleep(x) usleep((int)((x) * 1000000))
using namespace std;
class vprinter{
public:
int printerfile;
struct termios com4;
int chrset;
string
description[2];
string
taxdesc1;
string
taxdesc2;
string
taxdesc3;
vprinter();
~vprinter();
void printtext(string);
void linefeed();
void reset();
void formfeed();
//magnify 0-3
void magnify(int);
//charset 0-3
void charset(int);
void cleartabs();
void tab();
//tabs column in chars and tab number 1-32
void tabs(string);
void receipt(bool, string, string, string, string, string,
string);
void margin(int);
void pagelength(int);
void feedlength();
void getReceiptDesc();
int status();
};
vprinter::vprinter(){
chrset = 0;
long DATABITS = CS8;
long STOPBITS = 1;
long PARITYON = 0;
long PARITY = 0;
char *dev = "/dev/ttyS3";
com4.c_cflag = DATABITS | STOPBITS |
PARITYON | PARITY | CLOCAL | CREAD | CRTSCTS;
com4.c_iflag
= IGNPAR;
com4.c_oflag = 0;
com4.c_lflag = 0; //ICANON;
com4.c_cc[VMIN]=1;
com4.c_cc[VTIME]=0;
printerfile = open(dev, O_RDWR |
O_NOCTTY | O_NDELAY) ;
if (printerfile < 0)
{
cout << "Failed
to open com4!";
exit(-1);
}
cfsetispeed(&com4, B115200);
cfsetospeed(&com4, B115200);
tcflush(printerfile, TCIFLUSH);
tcsetattr(printerfile,TCSANOW,&com4);
reset();
getReceiptDesc();
feedlength();
cout << "description[0]=" << description[0] << endl;
cout << "description[1]=" << description[1] << endl;
cout << "taxdesc1=" << taxdesc1 << endl;
cout << "taxdesc2=" << taxdesc2 << endl;
cout << "taxdesc3=" << taxdesc3 << endl;
}
vprinter::~vprinter(){
close(printerfile);
}
void vprinter::printtext(string str) {
write(printerfile,str.c_str(),str.length());
}
void vprinter::linefeed(){
char cmd[] = {10};
write(printerfile,cmd,sizeof(cmd));
}
void vprinter::reset(){
char cmd[] = {27, 64};
write(printerfile,cmd,sizeof(cmd));
}
void vprinter::formfeed(){
char cmd[] = {12};
write(printerfile,cmd,sizeof(cmd));
}
void vprinter::magnify(int x){
char cmd[] = {27,33,0};
switch(x){
case 1:
cmd[2] = 4 + chrset;
break;
case 2:
cmd[2] = 8 + chrset;
break;
case 3:
cmd[2]
= 12 + chrset;
break;
default:
cmd[2] = 0 + chrset;
break;
}
write(printerfile,cmd,sizeof(cmd));
}
void vprinter::charset(int x){
char cmd[] = {27,33,0};
switch(x){
case 1:
chrset = 1;
break;
case 2:
chrset = 2;
break;
case 3:
chrset = 3;
break;
default:
chrset = 0;
break;
}
cmd[2] = chrset;
write(printerfile,cmd,sizeof(cmd));
}
void vprinter::tab(){
char cmd[] = {9};
write(printerfile,cmd,sizeof(cmd));
}
void vprinter::margin(int margin){
char cmd[] = {29, 76, 0,
0};
cmd[2] = margin;
write(printerfile,cmd,sizeof(cmd));
}
void vprinter::pagelength(int lines){
char cmd[] = {27, 67, 0};
cmd[2] = lines;
write(printerfile,cmd,sizeof(cmd));
}
void vprinter::feedlength(){
char cmd[] = {27, 205, 2,
129, 100, 1};
write(printerfile,cmd,sizeof(cmd));
}
void vprinter::cleartabs(){
char cmd[] = {27, 68, 0};
write(printerfile,cmd,sizeof(cmd));
}
void vprinter::tabs(string
positions){
char cmd[35] = {0};
int pos;
int startpos = 0;
int endpos = positions.find(',',
0);
int count = 2;
cmd[0] = 27;
cmd[1] = 68;
if (endpos > 0){
while(true){
istringstream x(positions.substr(startpos, endpos - startpos));
x >> pos;
cmd[count]
= pos;
count++;
startpos = endpos + 1;
endpos = positions.find(',', startpos);
if (endpos <= 0){
istringstream x(positions.substr(startpos, positions.size()));
x >> pos;
cmd[count] = pos;
count+=2;
break;
}
}
}
else {
istringstream x(positions);
x >> pos;
cmd[count] = pos;
count+=2;
}
for (int i = 0;i<=count;i++){
}
write(printerfile,cmd,count);
}
void vprinter::receipt(bool purchase, string
total, string beforetax, string tax1, string tax2,
string tax3, string pan){
string cmd;
string
dollar1 = "$";
string
dollar2 = "$";
string
dollar3 = "$";
string
dollar4 = "$";
string
dollar5 = "$";
string
card = "Card #: ";
time_t t;
struct tm *vtm;
char timeTmp[256];
string dt;
t =
time(NULL);
vtm = localtime(&t);
strftime(timeTmp, sizeof(timeTmp), "%m/%d/%y %H:%M",vtm);
dt.append("Date
(MM/DD/YY): ");
dt.append(timeTmp);
dollar1.append(beforetax);
dollar2.append(tax1);
dollar3.append(tax2);
dollar4.append(tax3);
dollar5.append(total);
card.append(pan);
ifstream scriptfile("/etc/kiosk/ReceiptScript.txt");
if (!scriptfile)
{
cerr << "opening ReceiptScriptfile failed\n";
exit(1);
}
while(getline(scriptfile, cmd)){
if (cmd.substr(0,sizeof("print:")-1)
== "print:")
printtext(cmd.erase(0, sizeof("print:")-1));
if (cmd.substr(0,sizeof("linefeed")-1)
== "linefeed")
linefeed();
if (cmd.substr(0,sizeof("magnify:")-1)
== "magnify:"){
istringstream x(cmd.erase(0, sizeof("magnify:")-1));
int i;
x
>> i;
magnify(i);
}
if (cmd.substr(0,sizeof("tab")-1)
== "tab")
tab();
if (cmd.substr(0,sizeof("formfeed")-1) == "formfeed")
formfeed();
if (cmd.substr(0,sizeof("productdesc")-1) == "productdesc")
printtext((purchase ? description[0] : description[1]));
if (cmd.substr(0,sizeof("beforetax")-1) == "beforetax")
printtext(dollar1);
if (cmd.substr(0,sizeof("taxdesc1")-1)
== "taxdesc1")
printtext(taxdesc1);
if (cmd.substr(0,sizeof("taxdesc2")-1)
== "taxdesc2")
printtext(taxdesc2);
if (cmd.substr(0,sizeof("taxdesc3")-1)
== "taxdesc3")
printtext(taxdesc3);
if (cmd.substr(0,sizeof("tax1")-1)
== "tax1")
printtext(dollar2);
if (cmd.substr(0,sizeof("tax2")-1)
== "tax2")
printtext(dollar3);
if (cmd.substr(0,sizeof("tax3")-1)
== "tax3")
printtext(dollar4);
if (cmd.substr(0,sizeof("total")-1)
== "total")
printtext(dollar5);
if (cmd.substr(0,sizeof("date")-1)
== "date")
printtext(dt);
if (cmd.substr(0,sizeof("card")-1)
== "card")
printtext(card);
if (cmd.substr(0,sizeof("margin:")-1)
== "margin:"){
istringstream x(cmd.erase(0, sizeof("margin:")-1));
int i;
x >> i;
margin(i);
}
if (cmd.substr(0,sizeof("pagelength:")-1) == "pagelength:"){
istringstream x(cmd.erase(0, sizeof("pagelength:")-1));
int i;
x >> i;
pagelength(i);
}
if (cmd.substr(0,sizeof("charset:")-1)
== "charset:"){
istringstream x(cmd.erase(0, sizeof("charset:")-1));
int i;
x >> i;
charset(i);
}
if (cmd.substr(0,sizeof("cleartabs")-1) == "cleartabs")
cleartabs();
if (cmd.substr(0,sizeof("tabs:")-1)
== "tabs:"){
cmd = cmd.erase(0, sizeof("tabs:")-1);
tabs(cmd);
}
}
scriptfile.close();
}
void vprinter::getReceiptDesc(){
ifstream receiptfile("/etc/kiosk/ReceiptDesc.txt");
if (!receiptfile)
{
cerr << "opening receiptfile failed\n";
exit(1);
}
getline(receiptfile,
description[0]);
getline(receiptfile,
description[1]);
getline(receiptfile, taxdesc1);
getline(receiptfile, taxdesc2);
getline(receiptfile, taxdesc3);
receiptfile.close();
description[0].erase(0,sizeof("Description1:")-1);
description[1].erase(0,sizeof("Description2:")-1);
taxdesc1.erase(0,sizeof("Taxdesc1:")-1);
taxdesc2.erase(0,sizeof("Taxdesc2:")-1);
taxdesc3.erase(0,sizeof("Taxdesc3:")-1);
}
int vprinter::status()
{
int i,x,y,tseconds;
int b[3]= {0};
struct timespec tstart, tstop;
fcntl(printerfile, F_SETFL,
FNDELAY);
cout << "Checking
Printer Status" << endl;
clock_gettime(CLOCK_REALTIME, &tstart);
while(!(b[0] && b[1] && b[2])){
clock_gettime(CLOCK_REALTIME, &tstop);
tseconds = tstop.tv_sec - tstart.tv_sec;
if(tseconds > 10)
break;
for (i = 0; i<3; i++){
char cmd[256] = {29, 97};
write(printerfile,cmd,sizeof(cmd));
usleep(1);
bzero(cmd, 256);
read(printerfile,cmd,sizeof(cmd));
b[i] = (int) cmd[0];
}
}
fcntl(printerfile, F_SETFL, 0);
cout << "b[0]=" << b[0] << " b[1]=" << b[1] << " b[2]=" << b[2] << endl;
x
= b[0];
y
= x | 127;
if (y == 255)
cout << "On-line" << endl;
y
= x | 191;
if (y == 255){
cout << "Paper
Out" << endl;
return(1);
}
y
= x | 223;
if (y == 255){
cout << "Low
Paper" << endl;
return(2);
}
y
= x | 239;
if (y == 255){
cout << "Paper
Jam" << endl;
return(3);
}
y
= x | 247;
if (y == 255)
cout << "Printout
Lost" << endl;
y
= x | 251;
if (y == 255)
cout << "Temperature
Alert" << endl;
return(0);
}
Copyright © 2013 ProTechs-Online.com; All rights reserved.