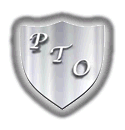
ProTech-Online.com
This is a C# code example of a Outlook 2010 Add-in Windows Forms.
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Windows.Forms;
using System.Data.SqlClient;
using Office = Microsoft.Office.Core;
using RibbonStuff = Microsoft.Office.Tools.Ribbon;
using Outlook = Microsoft.Office.Interop.Outlook;
namespace ChadSytelineOutlookAddIn
{
public partial class frmSL_Export : Form
{
private string control_id;
private Outlook.Inspector current_Inspector;
private Outlook.MailItem CurrentMailItem;
private string PR_SMTP_ADDRESS;
private static SqlConnection testsite_con = new SqlConnection(ChadSytelineOutlookAddIn.Properties.Settings.Default.testsiteConnectionString);
private static SqlConnection testsite3_con = new SqlConnection(ChadSytelineOutlookAddIn.Properties.Settings.Default.testsite3ConnectionString);
private static SqlConnection testsite2_con = new SqlConnection(ChadSytelineOutlookAddIn.Properties.Settings.Default.testsite2ConnectionString);
private SqlCommand testsite_cmd = new SqlCommand("Chad._chad_outlook_addin_autofind", testsite_con);
private SqlCommand testsite_cmd2 = new SqlCommand("Chad._chad_outlook_addin_search", testsite_con);
private SqlCommand testsite_cmd3 = new SqlCommand("Chad._chad_outlook_addin_search_incidents", testsite_con);
private SqlCommand testsite_cmd4 = new SqlCommand("Chad._chad_outlook_addin_export_cust_interaction", testsite_con);
private SqlCommand testsite_cmd5 = new SqlCommand("Chad._chad_outlook_addin_incident_shipto", testsite_con);
private SqlCommand testsite_cmd6 = new SqlCommand("Chad._chad_outlook_addin_export_incident", testsite_con);
private string tmp_site;
private string tmp_contact_type;
private string tmp_pci_num;
private string tmp_custseq;
private Outlook.Items sentitems;
public frmSL_Export(Outlook.Inspector currentInspector, string ControlID)
{InitializeComponent();
ckbIncidents.Checked = false;
control_id = ControlID;
current_Inspector = currentInspector;
CurrentMailItem = (Outlook.MailItem)currentInspector.CurrentItem;
PR_SMTP_ADDRESS = @"http://schemas.microsoft.com/mapi/proptag/0x39FE001E";
testsite_cmd.CommandType = CommandType.StoredProcedure;
testsite_cmd2.CommandType = CommandType.StoredProcedure;
testsite_cmd3.CommandType = CommandType.StoredProcedure;
testsite_cmd4.CommandType = CommandType.StoredProcedure;
testsite_cmd5.CommandType = CommandType.StoredProcedure;
testsite_cmd6.CommandType = CommandType.StoredProcedure;
sentitems =
CurrentMailItem.Session.GetDefaultFolder(Outlook.OlDefaultFolders.olFolderSentMail).Items;
sentitems.ItemAdd += SentItems_ItemAdd;
testsite_cmd.Parameters.Add("@EmailAddress", SqlDbType.NVarChar,
60);
testsite_cmd.Parameters.Add("@Site", SqlDbType.NVarChar, 10);
testsite_cmd2.Parameters.Add("@SearchText", SqlDbType.NVarChar,
60);
testsite_cmd2.Parameters.Add("@Site", SqlDbType.NVarChar, 10);
testsite_cmd3.Parameters.Add("@SearchText", SqlDbType.NVarChar,
60);
testsite_cmd3.Parameters.Add("@Site", SqlDbType.NVarChar, 10);
testsite_cmd4.Parameters.Add("@SL_SITE", SqlDbType.NVarChar, 10);
testsite_cmd4.Parameters.Add("@SL_CUSTNUM", SqlDbType.NVarChar, 7);
testsite_cmd4.Parameters.Add("@SL_SEQNUM", SqlDbType.Int);
testsite_cmd4.Parameters.Add("@SL_CONTACT_DATE", SqlDbType.DateTime);
testsite_cmd4.Parameters.Add("@SL_TOPIC", SqlDbType.NVarChar, 15);
testsite_cmd4.Parameters.Add("@SL_TYPE", SqlDbType.NChar, 1);
testsite_cmd4.Parameters.Add("@SL_EMAIL_STATUS", SqlDbType.NChar, 1);
testsite_cmd4.Parameters.Add("@SL_INCOMING", SqlDbType.TinyInt);
testsite_cmd4.Parameters.Add("@SL_NOTES", SqlDbType.NVarChar);
testsite_cmd4.Parameters.Add("@SL_ENTERED_BY", SqlDbType.NVarChar, 60);
testsite_cmd4.Parameters.Add("@SL_DESC", SqlDbType.NVarChar);
testsite_cmd4.Parameters.Add("@SL_INT_ID", SqlDbType.Decimal);
testsite_cmd4.Parameters["@SL_INT_ID"].Direction
= ParameterDirection.Output;
testsite_cmd5.Parameters.Add("@SL_SITE", SqlDbType.NVarChar, 10);
testsite_cmd5.Parameters.Add("@INC_NUM", SqlDbType.NVarChar, 10);
testsite_cmd6.Parameters.Add("@SL_SITE", SqlDbType.NVarChar, 10);
testsite_cmd6.Parameters.Add("@INC_NUM", SqlDbType.NVarChar, 10);
testsite_cmd6.Parameters.Add("@SL_DESC", SqlDbType.NVarChar);
testsite_cmd6.Parameters.Add("@SL_NOTES", SqlDbType.NText);
}
private void frmSL_Export_Load(object sender, EventArgs e)
{
this.Location = new Point((Screen.PrimaryScreen.WorkingArea.Width - this.Width) / 2,
(Screen.PrimaryScreen.WorkingArea.Height - this.Height) / 2);
}
private void btnAutoFind_Click(object sender, EventArgs e)
{
string SenderEmailAddress = "";
if (Results_ds.Tables["Results"].Rows.Count > 0)
Results_ds.Tables["Results"].Clear();
switch(control_id)
{
case "btnExportRead":
if (CurrentMailItem.Parent.FolderPath.Contains("Sent Items"))
{
Outlook.Recipients recips = CurrentMailItem.Recipients;
foreach (Outlook.Recipient recip in recips)
{
Outlook.PropertyAccessor testsite3 = recip.PropertyAccessor;
string smtpAddress = testsite3.GetProperty(PR_SMTP_ADDRESS).ToString();
testsite_cmd.Parameters["@EmailAddress"].Value
= smtpAddress;
if (rbtntestsite.Checked)
testsite_cmd.Parameters["@Site"].Value = "testsite";
if (rbtntestsite2.Checked)
testsite_cmd.Parameters["@Site"].Value
= "testsite2";
if (rbtntestsite3.Checked)
testsite_cmd.Parameters["@Site"].Value
= "testsite3";
if (!rbtntestsite.Checked && !rbtntestsite2.Checked && !rbtntestsite3.Checked)
testsite_cmd.Parameters["@Site"].Value
= null;
testsite_con.Open();
SqlDataReader reader = testsite_cmd.ExecuteReader();
while (reader.Read())
{
Results_ds.Tables["Results"].Rows.Add(
reader[0].ToString()
, reader[1].ToString()
, reader[2].ToString()
, reader[3].ToString()
, reader[4].ToString()
, reader[5].ToString()
, reader[6].ToString()
, reader[7].ToString()
, reader[8].ToString());
}
reader.Close();
testsite_con.Close();
}
}
else
{
if (CurrentMailItem.SenderEmailType == "EX")
{
Outlook.AddressEntry tmp_sender =
CurrentMailItem.Sender;
if (tmp_sender != null)
{
//Now we have an AddressEntry representing the Sender
if (tmp_sender.AddressEntryUserType == Outlook.OlAddressEntryUserType.olExchangeUserAddressEntry
|| tmp_sender.AddressEntryUserType == Outlook.OlAddressEntryUserType.olExchangeRemoteUserAddressEntry)
{
//Use the ExchangeUser object PrimarySMTtestsite3ddress
Outlook.ExchangeUser exchUser = tmp_sender.GetExchangeUser();
if (exchUser != null)
{
SenderEmailAddress = exchUser.PrimarySmtpAddress;
}
}
else
{
SenderEmailAddress = tmp_sender.PropertyAccessor.GetProperty(PR_SMTP_ADDRESS);
}
}
}
else if (CurrentMailItem.SenderEmailAddress != null)
{
SenderEmailAddress = CurrentMailItem.SenderEmailAddress;
}
testsite_cmd.Parameters["@EmailAddress"].Value
= SenderEmailAddress;
if (rbtntestsite.Checked)
testsite_cmd.Parameters["@Site"].Value = "testsite";
if (rbtntestsite2.Checked)
testsite_cmd.Parameters["@Site"].Value = "testsite2";
if (rbtntestsite3.Checked)
testsite_cmd.Parameters["@Site"].Value = "testsite3";
if (!rbtntestsite.Checked && !rbtntestsite2.Checked && !rbtntestsite3.Checked)
testsite_cmd.Parameters["@Site"].Value = null;
testsite_con.Open();
SqlDataReader reader = testsite_cmd.ExecuteReader();
while (reader.Read())
{
Results_ds.Tables["Results"].Rows.Add(
reader[0].ToString()
, reader[1].ToString()
, reader[2].ToString()
, reader[3].ToString()
, reader[4].ToString()
, reader[5].ToString()
, reader[6].ToString()
, reader[7].ToString()
, reader[8].ToString());
}
reader.Close();
testsite_con.Close();
}
break;
case "btnExportNew":
Outlook.Recipients recips1 = CurrentMailItem.Recipients;
foreach (Outlook.Recipient recip2 in recips1)
{
Outlook.PropertyAccessor testsite3 =
recip2.PropertyAccessor;
string smtpAddress = testsite3.GetProperty(PR_SMTP_ADDRESS).ToString();
testsite_cmd.Parameters["@EmailAddress"].Value
= smtpAddress;
if (rbtntestsite.Checked)
testsite_cmd.Parameters["@Site"].Value = "testsite";
if (rbtntestsite2.Checked)
testsite_cmd.Parameters["@Site"].Value = "testsite2";
if (rbtntestsite3.Checked)
testsite_cmd.Parameters["@Site"].Value = "testsite3";
if (!rbtntestsite.Checked && !rbtntestsite2.Checked && !rbtntestsite3.Checked)
testsite_cmd.Parameters["@Site"].Value = null;
testsite_con.Open();
SqlDataReader reader2 = testsite_cmd.ExecuteReader();
while (reader2.Read())
{
Results_ds.Tables["Results"].Rows.Add(
reader2[0].ToString()
, reader2[1].ToString()
, reader2[2].ToString()
, reader2[3].ToString()
, reader2[4].ToString()
, reader2[5].ToString()
, reader2[6].ToString()
, reader2[7].ToString()
, reader2[8].ToString());
}
reader2.Close();
testsite_con.Close();
}
break;
}
Results_ds.AcceptChanges();
dgvResults.AutoResizeColumns();
dgvResults.Refresh();
}
private void tbSearch_TextChanged(object sender, EventArgs e)
{
if (Results_ds.Tables["Results"].Rows.Count > 0)
Results_ds.Tables["Results"].Clear();
if (tbSearch.Text != "")
{
if (ckbIncidents.Checked)
{
testsite_cmd3.Parameters["@SearchText"].Value = tbSearch.Text.Replace("%", "");
if (rbtntestsite.Checked)
testsite_cmd3.Parameters["@Site"].Value = "testsite";
if (rbtntestsite2.Checked)
testsite_cmd3.Parameters["@Site"].Value = "testsite2";
if (rbtntestsite3.Checked)
testsite_cmd3.Parameters["@Site"].Value = "testsite3";
if (!rbtntestsite.Checked && !rbtntestsite2.Checked && !rbtntestsite3.Checked)
testsite_cmd3.Parameters["@Site"].Value = null;
testsite_con.Open();
SqlDataReader reader = testsite_cmd3.ExecuteReader();
while (reader.Read())
{
Results_ds.Tables["Results"].Rows.Add(
reader[0].ToString()
, reader[1].ToString()
, reader[2].ToString()
, reader[3].ToString()
, reader[4].ToString()
, reader[5].ToString()
, reader[6].ToString()
, reader[7].ToString()
, reader[8].ToString());
}
reader.Close();
testsite_con.Close();
}
else
{
testsite_cmd2.Parameters["@SearchText"].Value = tbSearch.Text.Replace("%", "");
if (rbtntestsite.Checked)
testsite_cmd2.Parameters["@Site"].Value = "testsite";
if (rbtntestsite2.Checked)
testsite_cmd2.Parameters["@Site"].Value = "testsite2";
if (rbtntestsite3.Checked)
testsite_cmd2.Parameters["@Site"].Value = "testsite3";
if (!rbtntestsite.Checked && !rbtntestsite2.Checked && !rbtntestsite3.Checked)
testsite_cmd2.Parameters["@Site"].Value = null;
testsite_con.Open();
SqlDataReader reader = testsite_cmd2.ExecuteReader();
while (reader.Read())
{
Results_ds.Tables["Results"].Rows.Add(
reader[0].ToString()
, reader[1].ToString()
, reader[2].ToString()
, reader[3].ToString()
, reader[4].ToString()
, reader[5].ToString()
, reader[6].ToString()
, reader[7].ToString()
, reader[8].ToString());
}
reader.Close();
testsite_con.Close();
}
Results_ds.AcceptChanges();
dgvResults.AutoResizeColumns();
dgvResults.Refresh();
}
}
private void ckbIncidents_CheckedChanged(object sender, EventArgs e)
{
btnAutoFind.Enabled = ckbIncidents.Checked ? false : true;
string tmp = this.tbSearch.Text;
this.tbSearch.Text = "";
this.tbSearch.Text = tmp;
}
private void dgvResults_CellDoubleClick(object sender, DataGridViewCellEventArgs e)
{
string site, contact_type, pci_num, seq;
site = dgvResults.SelectedCells[0].Value.ToString();
contact_type = dgvResults.SelectedCells[1].Value.ToString();
pci_num = dgvResults.SelectedCells[7].Value.ToString();
seq = dgvResults.SelectedCells[8].Value.ToString();
switch (control_id)
{
case "btnExportRead":
switch (contact_type)
{
case "Customer":
ExportCustomerRead(site, pci_num, seq);
break;
//case "Prospect":
// ExportProspectRead(site, pci_num);
// break;
case "Incident":
ExportIncidentRead(site, pci_num);
break;
}
this.Close();
break;
case "btnExportNew":
switch (contact_type)
{
case "Customer":
ExportCustomerNew(site, contact_type, pci_num, seq);
//this.DialogResult = System.Windows.Forms.DialogResult.Yes;
break;
//case "Prospect":
// ExporttestsiteProspectNew(site, pci_num, seq);
// break;
case "Incident":
ExportIncidentNew(site, contact_type, pci_num);
//this.DialogResult = System.Windows.Forms.DialogResult.Yes;
break;
}
break;
}
}
private void ExportCustomerRead(string site, string custnum, string custseq)
{
testsite_con.Open();
testsite_cmd4.Parameters["@SL_SITE"].Value
= site;
testsite_cmd4.Parameters["@SL_CUSTNUM"].Value
= custnum;
testsite_cmd4.Parameters["@SL_SEQNUM"].Value
= custseq;
testsite_cmd4.Parameters["@SL_TOPIC"].Value
= "OLI Customer";
testsite_cmd4.Parameters["@SL_TYPE"].Value
= "E";
if (CurrentMailItem.Parent.FolderPath.Contains("Sent Items"))
{
testsite_cmd4.Parameters["@SL_CONTACT_DATE"].Value
= CurrentMailItem.SentOn;
testsite_cmd4.Parameters["@SL_INCOMING"].Value
= 0;
}
else
{
testsite_cmd4.Parameters["@SL_CONTACT_DATE"].Value
= CurrentMailItem.ReceivedTime;
testsite_cmd4.Parameters["@SL_INCOMING"].Value
= 1;
}
string senderEmail = "";
if (CurrentMailItem.SenderEmailType == "EX")
{
senderEmail =
CurrentMailItem.Sender.PropertyAccessor.GetProperty(PR_SMTP_ADDRESS).ToString();
}
else if (CurrentMailItem.SenderEmailType == "SMTP")
{
senderEmail = CurrentMailItem.SenderEmailAddress;
}
if(CurrentMailItem.BodyFormat == Outlook.OlBodyFormat.olFormatHTML)
testsite_cmd4.Parameters["@SL_NOTES"].Value
= "From: " +
"<a href=\"mailto:" + senderEmail + "\">" +
CurrentMailItem.SenderName + "</a><br>" +
"To: " + CurrentMailItem.To + "<br>" +
"CC: " + CurrentMailItem.CC + "<br><br>" +
"Subject: " + CurrentMailItem.Subject + "<br><br>" +
CurrentMailItem.HTMLBody;
else
testsite_cmd4.Parameters["@SL_NOTES"].Value
= "From: " +
"<" + senderEmail + ">" +
CurrentMailItem.SenderName + "\r\n" +
"To: " + CurrentMailItem.To + "\r\n" +
"CC: " + CurrentMailItem.CC + "\r\n\r\n" +
"Subject: " + CurrentMailItem.Subject + "\r\n\r\n" +
CurrentMailItem.Body;
testsite_cmd4.Parameters["@SL_DESC"].Value
= CurrentMailItem.Subject;
testsite_cmd4.Parameters["@SL_ENTERED_BY"].Value
= Globals.ThisAddIn.username;
testsite_cmd4.ExecuteNonQuery();
switch (site)
{
case "testsite":
CurrentMailItem.SaveAs(@"\\chad1\SL_Imported_Files\Customer\OLI\testsite\SL_Int_" + testsite_cmd4.Parameters["@SL_INT_ID"].Value
+ ".msg");
break;
case "testsite2":
CurrentMailItem.SaveAs(@"\\chad1\SL_Imported_Files\Customer\OLI\testsite2\SL_Int_" + testsite_cmd4.Parameters["@SL_INT_ID"].Value
+ ".msg");
break;
case "testsite3":
CurrentMailItem.SaveAs(@"\\chad1\SL_Imported_Files\Customer\OLI\testsite3\SL_Int_" + testsite_cmd4.Parameters["@SL_INT_ID"].Value
+ ".msg");
break;
}
testsite_con.Close();
}
private void ExportProspectRead(string site, string custnum)
{
}
private void ExportIncidentRead(string site, string incident)
{
string interaction_id, custnum = "", custseq = "";
testsite_con.Open();
testsite_cmd5.Parameters["@SL_SITE"].Value
= site;
testsite_cmd5.Parameters["@INC_NUM"].Value
= incident;
SqlDataReader reader =
testsite_cmd5.ExecuteReader();
while (reader.Read())
{
custnum = reader[0].ToString();
custseq = reader[1].ToString();
}
reader.Close();
testsite_con.Close();
testsite_con.Open();
testsite_cmd4.Parameters["@SL_SITE"].Value
= site;
testsite_cmd4.Parameters["@SL_CUSTNUM"].Value
= custnum;
testsite_cmd4.Parameters["@SL_SEQNUM"].Value = custseq;
testsite_cmd4.Parameters["@SL_TOPIC"].Value
= "OLI Incident";
testsite_cmd4.Parameters["@SL_TYPE"].Value
= "E";
if (CurrentMailItem.Parent.FolderPath.Contains("Sent Items"))
{
testsite_cmd4.Parameters["@SL_CONTACT_DATE"].Value
= CurrentMailItem.SentOn;
testsite_cmd4.Parameters["@SL_INCOMING"].Value
= 0;
}
else
{
testsite_cmd4.Parameters["@SL_CONTACT_DATE"].Value
= CurrentMailItem.ReceivedTime;
testsite_cmd4.Parameters["@SL_INCOMING"].Value
= 1;
}
string senderEmail = "";
if (CurrentMailItem.SenderEmailType == "EX")
{
senderEmail =
CurrentMailItem.Sender.PropertyAccessor.GetProperty(PR_SMTP_ADDRESS).ToString();
}
else if (CurrentMailItem.SenderEmailType == "SMTP")
{
senderEmail = CurrentMailItem.SenderEmailAddress;
}
if (CurrentMailItem.BodyFormat == Outlook.OlBodyFormat.olFormatHTML)
testsite_cmd4.Parameters["@SL_NOTES"].Value
= "Incident: " + incident + "<br>" +
"From: " + "<a href=\"mailto:" + senderEmail + "\">" +
CurrentMailItem.SenderName + "</a><br>" +
"To: " + CurrentMailItem.To + "<br>" +
"CC: " + CurrentMailItem.CC + "<br><br>" +
"Subject: " + CurrentMailItem.Subject + "<br><br>" +
CurrentMailItem.HTMLBody;
else
testsite_cmd4.Parameters["@SL_NOTES"].Value
= "Incident: " + incident + "\r\n" +
"From: " + "<" + senderEmail + ">" +
CurrentMailItem.SenderName + "\r\n" +
"To: " + CurrentMailItem.To + "\r\n" +
"CC: " + CurrentMailItem.CC + "\r\n\r\n" +
"Subject: " + CurrentMailItem.Subject + "\r\n\r\n" +
CurrentMailItem.Body;
testsite_cmd4.Parameters["@SL_DESC"].Value
= CurrentMailItem.Subject;
testsite_cmd4.Parameters["@SL_ENTERED_BY"].Value
= Globals.ThisAddIn.username;
testsite_cmd4.ExecuteNonQuery();
interaction_id = testsite_cmd4.Parameters["@SL_INT_ID"].Value.ToString();
switch (site)
{
case "testsite":
CurrentMailItem.SaveAs(@"\\chad1\SL_Imported_Files\Customer\OLI\testsite\SL_Int_" + interaction_id + ".msg");
break;
case "testsite2":
CurrentMailItem.SaveAs(@"\\chad1\SL_Imported_Files\Customer\OLI\testsite2\SL_Int_" + interaction_id + ".msg");
break;
case "testsite3":
CurrentMailItem.SaveAs(@"\\chad1\SL_Imported_Files\Customer\OLI\testsite3\SL_Int_" + interaction_id + ".msg");
break;
}
testsite_con.Close();
testsite_con.Open();
testsite_cmd6.Parameters["@SL_SITE"].Value
= site;
testsite_cmd6.Parameters["@INC_NUM"].Value
= incident;
testsite_cmd6.Parameters["@SL_DESC"].Value
= DateTime.Now + " (" + Globals.ThisAddIn.username.Replace("Chad/", "") +
") " + CurrentMailItem.Subject;
if (CurrentMailItem.BodyFormat == Outlook.OlBodyFormat.olFormatHTML)
testsite_cmd6.Parameters["@SL_NOTES"].Value
= "Interaction: " + interaction_id + "<br>" +
"From: " + "<a href=\"mailto:" + senderEmail + "\">" +
CurrentMailItem.SenderName + "</a><br>" +
"To: " + CurrentMailItem.To + "<br>" +
"CC: " + CurrentMailItem.CC + "<br><br>" +
"Subject: " + CurrentMailItem.Subject + "<br><br>" +
CurrentMailItem.HTMLBody;
else
testsite_cmd6.Parameters["@SL_NOTES"].Value
= "Interaction: " + interaction_id + "\r\n" +
"From: " + "<" + senderEmail + ">" +
CurrentMailItem.SenderName + "\r\n" +
"To: " + CurrentMailItem.To + "\r\n" +
"CC: " + CurrentMailItem.CC + "\r\n\r\n" +
"Subject: " + CurrentMailItem.Subject + "\r\n\r\n" +
CurrentMailItem.Body;
testsite_cmd6.ExecuteNonQuery();
testsite_con.Close();
}
private void ExportCustomerNew(string site, string contact_type, string pci_num, string custseq)
{
tmp_site = site;
tmp_contact_type = contact_type;
tmp_pci_num = pci_num;
tmp_custseq = custseq;
Outlook.MailItem tmp_mail = CurrentMailItem.Copy();
tmp_mail.Send();
}
private void ExporttestsiteProspectNew(string site, string custnum, string custseq)
{
}
private void ExportIncidentNew(string site, string contact_type, string pci_num)
{
tmp_site = site;
tmp_contact_type = contact_type;
tmp_pci_num = pci_num;
Outlook.MailItem tmp_mail = CurrentMailItem.Copy();
tmp_mail.Send();
}
private void rbtntestsite_CheckedChanged(object sender, EventArgs e)
{
string tmp = this.tbSearch.Text;
this.tbSearch.Text = "";
this.tbSearch.Text = tmp;
}
private void rbtntestsite2_CheckedChanged(object sender, EventArgs e)
{
string tmp = this.tbSearch.Text;
this.tbSearch.Text = "";
this.tbSearch.Text = tmp;
}
private void rbtntestsite3_CheckedChanged(object sender, EventArgs e)
{
string tmp = this.tbSearch.Text;
this.tbSearch.Text = "";
this.tbSearch.Text = tmp;
}
public void SentItems_ItemAdd(object Item)
{
Outlook.MailItem SentMailItem;
SentMailItem = (Outlook.MailItem)Item;
switch (tmp_contact_type)
{
case "Customer":
testsite_con.Open();
testsite_cmd4.Parameters["@SL_SITE"].Value
= tmp_site;
testsite_cmd4.Parameters["@SL_CUSTNUM"].Value
= tmp_pci_num;
testsite_cmd4.Parameters["@SL_SEQNUM"].Value
= tmp_custseq;
testsite_cmd4.Parameters["@SL_TOPIC"].Value
= "OLI Customer";
testsite_cmd4.Parameters["@SL_TYPE"].Value = "E";
testsite_cmd4.Parameters["@SL_CONTACT_DATE"].Value
= DateTime.Now;
testsite_cmd4.Parameters["@SL_INCOMING"].Value
= 0;
if (CurrentMailItem.BodyFormat == Outlook.OlBodyFormat.olFormatHTML)
testsite_cmd4.Parameters["@SL_NOTES"].Value = "From: " +
"<a href=\"mailto:" + SentMailItem.Sender.PropertyAccessor.GetProperty(PR_SMTP_ADDRESS).ToString()
+ "\">" +
SentMailItem.SenderName + "</a><br>" +
"To: " + SentMailItem.To + "<br>" +
"CC: " +
SentMailItem.CC + "<br><br>" +
"Subject: " + SentMailItem.Subject + "<br><br>" +
SentMailItem.HTMLBody;
else
testsite_cmd4.Parameters["@SL_NOTES"].Value = "From: " +
"<" + SentMailItem.Sender.PropertyAccessor.GetProperty(PR_SMTP_ADDRESS).ToString()
+ ">" +
SentMailItem.SenderName + "\r\n" +
"To: " + SentMailItem.To + "\r\n" +
"CC: " +
SentMailItem.CC + "\r\n\r\n" +
"Subject: " + SentMailItem.Subject + "\r\n\r\n" +
SentMailItem.Body;
testsite_cmd4.Parameters["@SL_DESC"].Value
= SentMailItem.Subject;
testsite_cmd4.Parameters["@SL_ENTERED_BY"].Value
= Globals.ThisAddIn.username;
testsite_cmd4.ExecuteNonQuery();
switch (tmp_site)
{
case "testsite":
SentMailItem.SaveAs(@"\\chad1\SL_Imported_Files\Customer\OLI\testsite\SL_Int_" + testsite_cmd4.Parameters["@SL_INT_ID"].Value
+ ".msg");
break;
case "testsite2":
SentMailItem.SaveAs(@"\\chad1\SL_Imported_Files\Customer\OLI\testsite2\SL_Int_" + testsite_cmd4.Parameters["@SL_INT_ID"].Value
+ ".msg");
break;
case "testsite3":
SentMailItem.SaveAs(@"\\chad1\SL_Imported_Files\Customer\OLI\testsite3\SL_Int_" + testsite_cmd4.Parameters["@SL_INT_ID"].Value
+ ".msg");
break;
}
testsite_con.Close();
break;
//case "Prospect":
// ExporttestsiteProspectNew(site, pci_num, seq);
// break;
case "Incident":
string interaction_id, custnum = "", custseq = "";
testsite_con.Open();
testsite_cmd5.Parameters["@SL_SITE"].Value
= tmp_site;
testsite_cmd5.Parameters["@INC_NUM"].Value
= tmp_pci_num;
SqlDataReader reader = testsite_cmd5.ExecuteReader();
while (reader.Read())
{
custnum = reader[0].ToString();
custseq = reader[1].ToString();
}
reader.Close();
testsite_con.Close();
testsite_con.Open();
testsite_cmd4.Parameters["@SL_SITE"].Value = tmp_site;
testsite_cmd4.Parameters["@SL_CUSTNUM"].Value
= custnum;
testsite_cmd4.Parameters["@SL_SEQNUM"].Value
= custseq;
testsite_cmd4.Parameters["@SL_TOPIC"].Value
= "OLI Incident";
testsite_cmd4.Parameters["@SL_TYPE"].Value
= "E";
testsite_cmd4.Parameters["@SL_CONTACT_DATE"].Value
= DateTime.Now;
testsite_cmd4.Parameters["@SL_INCOMING"].Value
= 0;
if (CurrentMailItem.BodyFormat == Outlook.OlBodyFormat.olFormatHTML)
testsite_cmd4.Parameters["@SL_NOTES"].Value = "Incident: " + tmp_pci_num + "<br>" +
"From: " + "<a href=\"mailto:" + SentMailItem.Sender.PropertyAccessor.GetProperty(PR_SMTP_ADDRESS).ToString()
+ "\">" +
SentMailItem.SenderName + "</a><br>" +
"To: " + SentMailItem.To + "<br>" +
"CC: " +
SentMailItem.CC + "<br><br>" +
"Subject: " + SentMailItem.Subject + "<br><br>" +
SentMailItem.HTMLBody;
else
testsite_cmd4.Parameters["@SL_NOTES"].Value = "Incident: " + tmp_pci_num + "\r\n" +
"From: " + "<" + SentMailItem.Sender.PropertyAccessor.GetProperty(PR_SMTP_ADDRESS).ToString()
+ ">" +
SentMailItem.SenderName + "\r\n" +
"To: " + SentMailItem.To + "\r\n" +
"CC: " +
SentMailItem.CC + "\r\n\r\n" +
"Subject: " + SentMailItem.Subject + "\r\n\r\n" +
SentMailItem.Body;
testsite_cmd4.Parameters["@SL_DESC"].Value
= SentMailItem.Subject;
testsite_cmd4.Parameters["@SL_ENTERED_BY"].Value
= Globals.ThisAddIn.username;
testsite_cmd4.ExecuteNonQuery();
interaction_id = testsite_cmd4.Parameters["@SL_INT_ID"].Value.ToString();
switch (tmp_site)
{
case "testsite":
SentMailItem.SaveAs(@"\\chad1\SL_Imported_Files\Customer\OLI\testsite\SL_Int_" + interaction_id + ".msg");
break;
case "testsite2":
SentMailItem.SaveAs(@"\\chad1\SL_Imported_Files\Customer\OLI\testsite2\SL_Int_" + interaction_id + ".msg");
break;
case "testsite3":
SentMailItem.SaveAs(@"\\chad1\SL_Imported_Files\Customer\OLI\testsite3\SL_Int_" + interaction_id + ".msg");
break;
}
testsite_con.Close();
testsite_con.Open();
testsite_cmd6 = new SqlCommand("Chad._chad_outlook_addin_export_incident", testsite_con);
testsite_cmd6.CommandType = System.Data.CommandType.StoredProcedure;
testsite_cmd6.Parameters.Add("@SL_SITE", SqlDbType.NVarChar, 10);
testsite_cmd6.Parameters.Add("@INC_NUM", SqlDbType.NVarChar, 10);
testsite_cmd6.Parameters.Add("@SL_DESC", SqlDbType.NVarChar);
testsite_cmd6.Parameters.Add("@SL_NOTES", SqlDbType.NText);
testsite_cmd6.Parameters["@SL_SITE"].Value
= tmp_site;
testsite_cmd6.Parameters["@INC_NUM"].Value
= tmp_pci_num;
testsite_cmd6.Parameters["@SL_DESC"].Value = DateTime.Now + "
(" + Globals.ThisAddIn.username.Replace("Chad/", "") +
") " + SentMailItem.Subject;
if (CurrentMailItem.BodyFormat == Outlook.OlBodyFormat.olFormatHTML)
testsite_cmd6.Parameters["@SL_NOTES"].Value = "Interaction: " + interaction_id + "<br>" +
"From: " + "<a href=\"mailto:" + SentMailItem.Sender.PropertyAccessor.GetProperty(PR_SMTP_ADDRESS).ToString()
+ "\">" +
SentMailItem.SenderName + "</a><br>" +
"To: " + SentMailItem.To + "<br>" +
"CC: " +
SentMailItem.CC + "<br><br>" +
"Subject: " + SentMailItem.Subject + "<br><br>" +
SentMailItem.HTMLBody;
else
testsite_cmd6.Parameters["@SL_NOTES"].Value = "Interaction: " + interaction_id + "\r\n" +
"From: " + "<" + SentMailItem.Sender.PropertyAccessor.GetProperty(PR_SMTP_ADDRESS).ToString()
+ ">" +
SentMailItem.SenderName + "\r\n" +
"To: " + SentMailItem.To + "\r\n" +
"CC: " +
SentMailItem.CC + "\r\n\r\n" +
"Subject: " + SentMailItem.Subject + "\r\n\r\n" +
SentMailItem.Body;
testsite_cmd6.ExecuteNonQuery();
testsite_con.Close();
break;
}
this.DialogResult = System.Windows.Forms.DialogResult.Yes;
this.Close();
}
private void frmSL_Export_FormClosed(object sender, FormClosedEventArgs e)
{
sentitems.ItemAdd -= SentItems_ItemAdd;
}
}
}
Copyright © 2013 ProTechs-Online.com; All rights reserved.