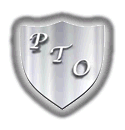
ProTech-Online.com
This is a C# code example of a Outlook 2010 Add-in Options Dialog.
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Drawing;
using System.Data;
using System.Linq;
using System.Text;
using System.Windows.Forms;
using System.Runtime.InteropServices;
using Outlook = Microsoft.Office.Interop.Outlook;
using System.Text.RegularExpressions;
namespace ChadSytelineOutlookAddIn
{
[ComVisible(true)]
public partial class ChadAddinOptions : UserControl, Outlook.PropertyPage
{
const int captionDispID = -518;
bool isDirty = false;
private Outlook.PropertyPageSite propertyPageSite = null;
public ChadAddinOptions()
{
InitializeComponent();
}
void Outlook.PropertyPage.Apply()
{
Globals.ThisAddIn.SytelineUsername = tbUsername.Text;
Globals.ThisAddIn.SytelineSavePath = tbSavePath.Text;
}
bool Outlook.PropertyPage.Dirty
{
get
{
return isDirty;
}
}
void Outlook.PropertyPage.GetPageInfo(ref string helpFile, ref int helpContext)
{
}
[DispId(captionDispID)]
public string PageCaption
{
get
{
return "Chad Syteline Outlook Add-in";
}
}
private void tbUsername_TextChanged(object sender, EventArgs e)
{
isDirty = true;
propertyPageSite.OnStatusChange();
}
private Outlook.PropertyPageSite GetPropertyPageSite()
{
Type myType = typeof(System.Object);
string assembly = Regex.Replace(myType.Assembly.CodeBase, "mscorlib.dll", "System.Windows.Forms.dll");
assembly = System.Text.RegularExpressions.Regex.Replace(assembly, "file:///", "");
assembly = System.Reflection.AssemblyName.GetAssemblyName(assembly).FullName;
Type unmanaged = Type.GetType(System.Reflection.Assembly.CreateQualifiedName(assembly, "System.Windows.Forms.UnsafeNativeMethods"));
Type oleObj = unmanaged.GetNestedType("IOleObject");
System.Reflection.MethodInfo mi = oleObj.GetMethod("GetClientSite");
object myppSite = mi.Invoke(this, null);
return (Outlook.PropertyPageSite)myppSite;
}
private void ChadAddinOptions_Load(object sender, EventArgs e)
{
propertyPageSite = GetPropertyPageSite();
tbUsername.Text = Globals.ThisAddIn.SytelineUsername;
tbSavePath.Text = Globals.ThisAddIn.SytelineSavePath;
}
}
}
Copyright © 2013 ProTechs-Online.com; All rights reserved.