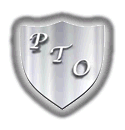
ProTech-Online.com
This is a C# code example of a Outlook 2010 Add-in Export Button.
using System;
using System.Collections.Generic;
using System.IO;
using System.Linq;
using System.Reflection;
using System.Runtime.InteropServices;
using System.Text;
using Office = Microsoft.Office.Core;
using RibbonStuff = Microsoft.Office.Tools.Ribbon;
using Outlook = Microsoft.Office.Interop.Outlook;
using System.Windows.Forms;
// TODO: Follow these steps to enable the Ribbon (XML)
item:
// 1: Copy the following code block
into the ThisAddin, ThisWorkbook,
or ThisDocument class.
// protected override Microsoft.Office.Core.IRibbonExtensibility CreateRibbonExtensibilityObject()
// {
// return new ChadRibbon();
// }
// 2. Create callback methods in the
"Ribbon Callbacks" region of this class to
handle user
// actions, such as clicking a button. Note: if you have exported this
Ribbon from the Ribbon designer,
// move your code from the event handlers to the callback methods and
modify the code to work with the
// Ribbon extensibility (RibbonX) programming
model.
// 3. Assign attributes to the control
tags in the Ribbon XML file to identify the appropriate callback methods in
your code.
// For more
information, see the Ribbon XML documentation in the Visual Studio Tools for
Office Help.
namespace ChadSytelineOutlookAddIn
{
[ComVisible(true)]
public class ChadRibbon : Office.IRibbonExtensibility
{
private Office.IRibbonUI ribbon;
public ChadRibbon()
{
}
#region IRibbonExtensibility Members
public string GetCustomUI(string ribbonID)
{
return GetResourceText("ChadSytelineOutlookAddIn.ChadRibbon.xml");
}
#endregion
#region Ribbon Callbacks
//Create callback methods here. For more information about adding callback methods, select
the Ribbon XML item in Solution Explorer and then press F1
public void Ribbon_Load(Office.IRibbonUI ribbonUI)
{
this.ribbon = ribbonUI;
}
#endregion
#region Helpers
private static string GetResourceText(string resourceName)
{
Assembly asm = Assembly.GetExecutingAssembly();
string[] resourceNames = asm.GetManifestResourceNames();
for (int i = 0; i < resourceNames.Length; ++i)
{
if (string.Compare(resourceName, resourceNames[i], StringComparison.OrdinalIgnoreCase)
== 0)
{
using (StreamReader resourceReader = new StreamReader(asm.GetManifestResourceStream(resourceNames[i])))
{
if (resourceReader != null)
{
return resourceReader.ReadToEnd();
}
}
}
}
return null;
}
#endregion
public void SL_Export(Office.IRibbonControl control)
{
Outlook.Inspector currentInspector = (Outlook.Inspector)control.Context;
if (currentInspector.CurrentItem.MessageClass == "IPM.Note")
{
Form SL_ExportForm = new frmSL_Export(currentInspector, control.Id);
DialogResult result = SL_ExportForm.ShowDialog();
if (result == DialogResult.Yes)
{
currentInspector.Close(Outlook.OlInspectorClose.olDiscard);
}
}
else
MessageBox.Show("Can't export this type of
message!", "Chad Add-in", MessageBoxButtons.OK, MessageBoxIcon.Information);
}
}
}
Copyright © 2013 ProTechs-Online.com; All rights reserved.