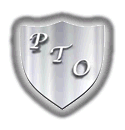
ProTech-Online.com
This is a C# code example of a Outlook 2010 Add-in adding the Options Page and Registry Keys.
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Xml.Linq;
using Outlook = Microsoft.Office.Interop.Outlook;
using Office = Microsoft.Office.Core;
using Microsoft.Win32;
using System.Security.AccessControl;
using System.Windows.Forms;
namespace ChadSytelineOutlookAddIn
{
public partial class ThisAddIn
{
private string user = Environment.UserDomainName + "\\" + Environment.UserName;
private RegistrySecurity rs = new RegistrySecurity();
public string SytelineSystem;
public string SytelineUsername;
public string SytelineSavePath;
private void ThisAddIn_Startup(object sender, System.EventArgs e)
{
((Outlook.ApplicationEvents_11_Event)Application).OptionsPagesAdd += new Outlook.ApplicationEvents_11_OptionsPagesAddEventHandler(Application_OptionsPagesAdd);
((Outlook.ApplicationEvents_10_Event)Application).Quit += new Outlook.ApplicationEvents_10_QuitEventHandler(ThisAddIn_Quit);
((Outlook.ApplicationEvents_11_Event)Application).Quit += new Outlook.ApplicationEvents_11_QuitEventHandler(ThisAddIn_Quit);
rs.AddAccessRule(new RegistryAccessRule(
user
,RegistryRights.FullControl
,InheritanceFlags.None
,PropagationFlags.None
,AccessControlType.Allow));
try
{
RegistryKey rk = Registry.CurrentUser.OpenSubKey(@"Software\Chad Machine
Inc.\Chad Syteline Outlook Addin");
if (rk == null)
{
rk = Registry.CurrentUser.CreateSubKey(@"Software\Chad Machine Inc.\Chad Syteline Outlook Addin",
RegistryKeyPermissionCheck.Default, rs);
rk.SetValue("Last_System", "testsite");
rk.SetValue("Username", "sa");
rk.SetValue("Path", @"\\chad1\Chad_drive\Syteline_Emails");
}
SytelineSystem = rk.GetValue("Last_System", "testsite").ToString();
SytelineUsername = rk.GetValue("Username", "sa").ToString();
SytelineSavePath = rk.GetValue("Path", @"\\chad1\Chad_drive\Syteline_Emails").ToString();
rk.Close();
}
catch (Exception ex)
{
MessageBox.Show("Error Occured:
" + ex.Message, "Chad
Syteline Outlook AddIn");
}
}
private void ThisAddIn_Shutdown(object sender, System.EventArgs e)
{
}
void ThisAddIn_Quit()
{
try
{
RegistryKey rk = Registry.CurrentUser.OpenSubKey(@"Software\Chad Machine
Inc.\Chad Syteline Outlook Addin",true);
rk.SetValue("Last_System", SytelineSystem);
rk.SetValue("Username", SytelineUsername);
rk.SetValue("Path", SytelineSavePath);
rk.Close();
}
catch (Exception ex)
{
MessageBox.Show("Error Occured:
" + ex.Message, "Chad
Syteline Outlook AddIn");
}
}
void Application_OptionsPagesAdd(Outlook.PropertyPages Pages)
{
Pages.Add(new ChadAddinOptions(), "");
}
#region VSTO
generated code
/// <summary>
/// Required method for Designer support - do not modify
/// the
contents of this method with the code editor.
/// </summary>
private void InternalStartup()
{
this.Startup += new System.EventHandler(ThisAddIn_Startup);
this.Shutdown += new System.EventHandler(ThisAddIn_Shutdown);
}
#endregion
}
}
Copyright © 2013 ProTechs-Online.com; All rights reserved.